はじめに
プログラミングが全然理解できていない、ど素人が書いたコードです。
一応、意図通りに動いているので公開しますが不具合等ありましたら、すみません。
データを比較する
この記事では、前編でAPIから取得したデータと中編でスプレッドシートに書き込んでおいた少し前のデータを比較し、今日のキル数などを算出していきます。
長かった解説も今回で最後です。もう少しだけお付き合いください。
事前準備
前回、スプレッドシートへ自動でAPIから取得したデータを保存することができたので、そのデータを昨日までのデータとして参照してみます。
今回はJSONとして利用するので、中編で作成したスプレッドシートをメニューのファイルからwebに公開しておいてください。
JSONに変換する
何も難しいことはありません、スプレッドシートのIDをこちらのURLに組み込むだけです。
https://spreadsheets.google.com/feeds/list/スプレッドシートのID/od6/public/values?alt=jsonスプレッドシートIDの後ろの「od6」はワークシートの順番になります。
2番目以降のワークシートを使いたい場合は、人によって変わったりもするので各自調べてみてください。
そのURLにアクセスすると、無事データがJSON化されていますがごちゃごちゃと見づらくわけがわからないと思うので、Chromeで拡張機能のJSON Viewerや整形してくれるサイトを利用して、ほしいデータがどう格納されているか確認してください。
私の場合だと、このようになっています。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
{ "version": "1.0", "encoding": "UTF-8", "feed": { "xmlns": "http://www.w3.org/2005/Atom", "xmlns$openSearch": "http://a9.com/-/spec/opensearchrss/1.0/", "xmlns$gsx": "http://schemas.google.com/spreadsheets/2006/extended", "id": { "$t": "https://spreadsheets.google.com/feeds/list/12PI1MWYdmWzpspcUtkhff1e2bnClzfWGWLvOWQeMgV0/od6/public/values" }, "title": { "type": "text", "$t": "Apex" }, "openSearch$totalResults": { "$t": "2" }, "openSearch$startIndex": { "$t": "1" }, "entry": [ { "category": [ { "scheme": "http://schemas.google.com/spreadsheets/2006", "term": "http://schemas.google.com/spreadsheets/2006#list" } ], "title": { "type": "text", "$t": "oboro" }, "content": { "type": "text", "$t": "kills: 7574, damage: 2145924, s4win: 84" }, "link": [ { "rel": "self", "type": "application/atom+xml", "href": "https://spreadsheets.google.com/feeds/list/12PI1MWYdmWzpspcUtkhff1e2bnClzfWGWLvOWQeMgV0/od6/public/values/cokwr" } ], "gsx$player": { "$t": "oboro" }, "gsx$kills": { "$t": "7574" }, "gsx$damage": { "$t": "2145924" }, "gsx$s4win": { "$t": "84" } }, ] } } |
PHPでシートを参照するコード
JSONがどう書かれているかもわかったので、PHPに組み込んでいきます。
1 2 3 4 5 6 7 |
// JSON化したスプレッドシートを読み込む $data = "JSON化したスプレッドシートのURL"; $json = file_get_contents($data); $json_decode = json_decode($json); // JSONデータ内の『entry』部分を複数取得して、postsに格納 $posts = $json_decode->feed->entry; |
このpostsからほしい情報、例としてキル数を抜き出してみます。
キル数は feed > entry > gsx$kills > t にあるのでこんな感じに指定してあげます。
1 2 3 |
foreach ($posts as $post) { $ytk[] = $post->{'gsx$kills'}->{'$t'}; } |
私の場合は昨日までのトータルキルって意味で $ytk という名前で管理しています。
あとは同じようにダメージと勝利数、同じデータ名の中でも最初のという意味で $i=0; も追加してあげます。
1 2 3 4 5 6 7 8 9 10 |
foreach ($posts as $post) { $ytk[] = $post->{'gsx$kills'}->{'$t'}; } foreach ($posts as $post) { $ytd[] = $post->{'gsx$damage'}->{'$t'}; } foreach ($posts as $post) { $ytw[] = $post->{'gsx$s4win'}->{'$t'}; } $i=0; |
現在の値から昨日の値の差分を算出
前編でAPIから取得した値からスプレッドシートから取得した値を引くことで、今日の差分の値が算出できます。
1 2 3 |
$todaykills = $tk - $ytk[$i]; $todaydamage = $td - $ytd[$i]; $todaywins = $ts4w - $ytw[$i]; |
そのままです。- (マイナス)してあげるだけです。
コードをまとめる
前編のAPIからデータを取得するコードと、今回のスプレッドシートからデータを取得、差分を算出するコードをまとめるとこのようになります。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
<?php { //API Key $header = array( "TRN-Api-Key:入手したAPI Key" ); //user情報取得 $ch = curl_init(); curl_setopt($ch,CURLOPT_URL,"https://public-api.tracker.gg/v2/apex/standard/profile/プラットフォーム/ユーザー名"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $header); $response = curl_exec($ch); $profile = json_decode($response, true); $tk = ($profile['data']['segments'][0]['stats']['kills']['value']); $td = ($profile["data"]["segments"][0]["stats"]["damage"]["value"]); $ts4w = ($profile["data"]["segments"][0]["stats"]["season4Wins"]["value"]); curl_close($ch); } { // JSON化したスプレッドシートを読み込む $data = "JSON化したスプレッドシートのURL"; $json = file_get_contents($data); $json_decode = json_decode($json); // JSONデータ内の『entry』部分を複数取得して、postsに格納 $posts = $json_decode->feed->entry; foreach ($posts as $post) { $ytk[] = $post->{'gsx$kills'}->{'$t'}; } foreach ($posts as $post) { $ytd[] = $post->{'gsx$damage'}->{'$t'}; } foreach ($posts as $post) { $ytw[] = $post->{'gsx$s4win'}->{'$t'}; } $i=0; $todaykills = $tk - $ytk[$i]; $todaydamage = $td - $ytd[$i]; $todaywins = $ts4w - $ytw[$i]; } ?> |
処理部分はこれにて全て完成です。
サイトとして公開してみる
処理部分は全て終わっているので、あとはhtmlとして出力してあげればサイトとして完成します。
簡単ですが、このようにしてみました。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
<?php { //API Key $header = array( "TRN-Api-Key:入手したAPI Key" ); //user情報取得 $ch = curl_init(); curl_setopt($ch,CURLOPT_URL,"https://public-api.tracker.gg/v2/apex/standard/profile/プラットフォーム/ユーザー名"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, $header); $response = curl_exec($ch); $profile = json_decode($response, true); $tk = ($profile['data']['segments'][0]['stats']['kills']['value']); $td = ($profile["data"]["segments"][0]["stats"]["damage"]["value"]); $ts4w = ($profile["data"]["segments"][0]["stats"]["season4Wins"]["value"]); curl_close($ch); } { // JSON化したスプレッドシートを読み込む $data = "JSON化したスプレッドシートのURL"; $json = file_get_contents($data); $json_decode = json_decode($json); // JSONデータ内の『entry』部分を複数取得して、postsに格納 $posts = $json_decode->feed->entry; foreach ($posts as $post) { $ytk[] = $post->{'gsx$kills'}->{'$t'}; } foreach ($posts as $post) { $ytd[] = $post->{'gsx$damage'}->{'$t'}; } foreach ($posts as $post) { $ytw[] = $post->{'gsx$s4win'}->{'$t'}; } $i=0; $todaykills = $tk - $ytk[$i]; $todaydamage = $td - $ytd[$i]; $todaywins = $ts4w - $ytw[$i]; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="ja" lang="ja"> <head> <meta http-equiv="Content-type" content="text/html; charset=UTF-8"> <title>Apex Stats</title> </head> <body> <header> </header> <main> <div class="apex_stats"> <h1>Apex Stats</h1> <?php //出力 echo '<p><span class="title">Season 4 Wins : </span><span class="value">'; echo number_format($ts4w); echo "</span></p>"; echo '<p><span class="title">Today's CHAMPION : </span><span class="value">'; echo number_format($todaywins); echo "</span></p>"; echo '<p><span class="title">Total Kills : </span><span class="value">'; echo number_format($tk); echo "</span></p>"; echo '<p><span class="title">Today's Kills : </span><span class="value">'; echo number_format($todaykills); echo "</span></p>"; echo '<p><span class="title">Total Damage : </span><span class="value">'; echo number_format($td); echo "</span></p>"; echo '<p><span class="title">Today's Damage : </span><span class="value">'; echo number_format($todaydamage); echo "</span></p>"; ?> </div> <footer> </footer> </main> </body> </html> |
オーバーレイとして使うには
配信時のオーバーレイとして使うには、リアルタイムで数値が変化していくように定期的にリロードさせてあげることが必要です。
方法はいろいろありますが、今回はjavascriptでリロードするようにしてみます。
1 2 3 4 5 6 7 |
<script type="text/javascript"> var timer = "180000"; //指定ミリ秒単位 function ReloadAddr(){ window.location.reload(); //ページをリロード } setTimeout(ReloadAddr, timer); </script> |
APIの負荷も考えて3分毎リロードするようにしてあります。
OBSなどのブラウザ機能で読み込んだあとは、cssでスタイルを整えたりマスクで要らないところを隠したりして使ってみてください。
完成サンプル
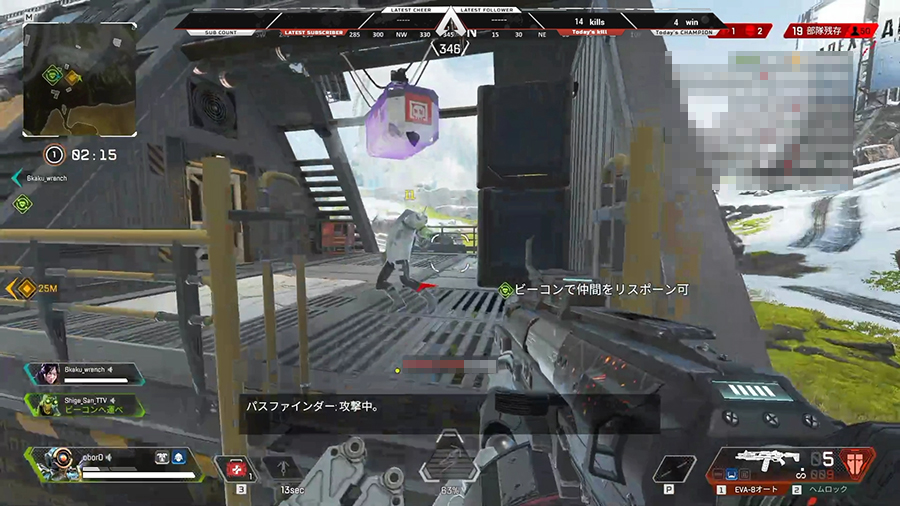
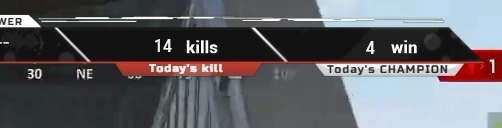
実際にオーバーレイさせてみると、こんな感じになります。
ごあいさつ
簡潔とはほど遠い長い解説になってしまいましたが、お付き合いありがとうございました。
某トラッカーサイトに配信用のウィジェットがあったのですが、レジェンドに紐づいてて、選んだレジェンドによっては不安定になるので今回これを作ってみました。
先人達のいろんな解説サイトからアイデアやコードをもらってきたので、統一性がなかったり知識不足で変な箇所もあるかと思いますが、参考にしてみてください。